view plain
view html
# Handler
The handler object is an object to get, set, delete, update and export defined CSS properties. You get this object from the controller object
```javascript
var h = CSSC(".className"); //get a handler object with all CSS objects are defined as .className
h = CSSC([".className1", ".className2"]); //get a handler object with .className1 and .className2
h = CSSC(/\.className[0-9]/); //get a handler obejct with objects matched to regular expression
h = CSSC(); //get a handler object with all defined CSS objects
```
## Methods
---
### .get()
**get** is a method to get CSS properties
```
.get([propertie[, returnAll]])
```
* *`propertie` \[optional\]* - A String with property name. If this value is not given, return this method an object with all properies of the Handler object
* *`returnAll` \[optional\]* - A Boolean. If true, the return value is an Array with all found properties; if false, the return value is the last definition of property in the Handler object (default: false)
**`Return value`** - Mixed -> Object, String or Array of Strings, depending on how the parameters were set
#### Example
```javascript
CSSC({
body: [{
margin: 10,
padding: 5,
},{
border: "1 solid #ccc",
padding: 7
}]
});
var val = CSSC("body").get("padding");
console.log(val);
/*
7px
*/
val = CSSC("body").get("padding", true);
console.log(JSON.stringify(val, true, 4));
/*
[
"5px",
"7px"
]
*/
val = CSSC("body").get();
console.log(JSON.stringify(val, true, 4));
/*
{
"body": [
{
"margin": "10px",
"padding": "5px"
},
{
"border": "1px solid #ccc",
"padding": "7px"
}
]
}
*/
```
---
### .set()
**set** is a method to set CSS properties
```
.set(toSet[, value])
```
* `toSet` - A property to set as String, an object to set with key-value pair, a function that returns the values to set, or Array containing an object or function with key-value.
* *`value` \[optional\]* - use this when `toSet` a String. A value to set as String/Integer/Float, a function that returns the values to set, an object to create a new CSS rule or an Array with objects to create new rules.
**`Return value`** - Handler object.
#### Example
```javascript
CSSC({
body: {
margin: 10,
padding: 5,
}
});
CSSC("body").set("border", "1 solid red");
console.log(CSSC.parse());
/*
body {
margin: 10px;
padding: 5px;
border: 1px solid red;
}
*/
CSSC("body").set({margin: 20, padding: 0});
console.log(CSSC.parse());
/*
body {
margin: 20px;
padding: 0px;
border: 1px solid red;
}
*/
CSSC("body").set(".newClass",{margin: "5 0 0 10", float: "left"});
console.log(CSSC.parse());
/*
body {
margin: 20px;
padding: 0px;
border: 1px solid red;
}
body .newClass {
margin: 5px 0px 0px 10px;
float: left;
}
*/
CSSC("body .newClass").set({border: "1 solid #ccc", "/.class1":{float: "none"}});
console.log(CSSC.parse());
/*
body {
margin: 20px;
padding: 0px;
border: 1px solid red;
}
body .newClass {
margin: 5px 0px 0px 10px;
float: left;
border: 1px solid #ccc;
}
body .newClass.class1 {
float: none;
}
*/
```
---
### .delete()
**delete** is a method to delete a CSS property or a CSS rule
```
.delete([property])
```
* *`property` \[optional\]* - A property name to delete. If this value not given, the method deletes the complete rule(s)
**`Return value`** - Handler object.
#### Example
```javascript
CSSC({
body: [{
margin: 10,
padding: 5,
},{
border: "1 solid #ccc",
padding: 7
}]
});
var parsed = CSSC.parse();
console.log(parsed);
/*
body {
margin: 10px;
padding: 5px;
}
body {
border: 1px solid #ccc;
padding: 7px;
}
*/
// delete property
CSSC("body").delete("padding");
parsed = CSSC.parse();
console.log(parsed);
/*
body {
margin: 10px;
}
body {
border: 1px solid #ccc;
}
*/
//delete rule
CSSC("body").first().delete();
parsed = CSSC.parse();
console.log(parsed);
/*
body {
border: 1px solid #ccc;
}
*/
```
---
### .update()
**update** is a method to update updatable properties or rules
```
.update()
```
**`Return value`** - Handler object.
#### Example
```javascript
CSSC({
'.updatable1': function(){
return {
width: Math.random()*100+100,
height: Math.random()*100+100,
};
},
'.updatable2': {
width: 20,
height: function(){ return Math.random()*10+10; }
}
});
var parsed = CSSC.parse();
console.log(parsed);
/*
.updatable1 {
width: 166.16px;
height: 147.66px;
}
.updatable2 {
width: 20px;
height: 16.13px;
}
*/
CSSC(".updatable1").update();
parsed = CSSC.parse();
console.log(parsed);
/*
.updatable1 {
width: 101.4px;
height: 143.95px;
}
.updatable2 {
width: 20px;
height: 16.13px;
}
*/
CSSC(/\.updatable[12]/).update();
parsed = CSSC.parse();
console.log(parsed);
/*
.updatable1 {
width: 117.66px;
height: 198.24px;
}
.updatable2 {
width: 20px;
height: 10.94px;
}
*/
```
---
### .export()
**export** is a method to export defined CSS as String, Object or Array
```
.export([exportType])
```
* *`exportType` \[optional\]* - String with export type (default: "object")
* *`"css"` - export as CSS String*
* *`"min"` - export as minified CSS String*
* *`"obj"` - export as JS-Object*
* *`"arr"` - export as array*
* *`"object"` - the same as "obj"*
* *`"objNMD"` - export as not multidimensional object*
* *`"array"` - the same as "arr"*
**`Return value`** - Mixed
#### Example
```javascript
CSSC({
body: {
margin: 1
},
p: {
width: 500,
margin: "auto",
"span.first": {
"font-size": 25
},
"@media screen and (max-width: 500px)": {
width: "100%"
}
}
});
var exportObject = CSSC("p").export(); // or CSSC.export("obj") or CSSC.export("object")
console.log(JSON.stringify(exportObject, true, 4));
/*
{
"p": {
"width": "500px",
"margin": "auto",
"span.first": {
"font-size": "25px"
},
"@media screen and (max-width: 500px)": {
"width": "100%"
}
}
}
*/
exportObject = CSSC("p").export("css");
console.log(exportObject);
/*
p {
width: 500px;
margin: auto;
}
*/
```
---
### .parse()
**parse** is a method to parse defined CSS. This method is identical to .export(CSSC.type_export.css) or .export(CSSC.type_export.min)
```
.parse([min])
```
* *`min` \[optional]\* - Boolean, if true return a minified CSS (default: false)
**`Return value`** - String with CSS
#### Example
```javascript
/*
this method returns the same result as .export("css") or .export("min");
*/
exportObject = CSSC("p").parse(true);
console.log(exportObject);
/*
p{width:500px;margin:auto;}
*/
```
---
### .pos()
**pos** is a method to get a Handler object with style element of the given position
```
.pos(position)
```
* `position` - Integer, the position of found style elements. If the position is negative, count the position from last.
**`Return value`** - Handler object with style element of the given position
#### Example
```javascript
CSSC({
p: [{
height: 100
},{
width: 500
},{
color: "green"
}]
});
var handler = CSSC("p");
console.log(handler.e.length);
/*
3
*/
console.log(handler.parse());
/*
p {
height: 100px;
}
p {
width: 500px;
}
p {
color: green;
}
*/
console.log(handler.pos(0).parse());
/*
p {
height: 100px;
}
*/
console.log(handler.pos(1).parse());
/*
p {
width: 500px;
}
*/
console.log(handler.pos(-1).parse());
/*
p {
color: green;
}
*/
```
---
### .first()
**first** is a method to get a Handler object with first style element. This method is equivalent to `.pos(0)`
```
.first()
```
**`Return value`** - Handler object with first style element
#### Example
```javascript
CSSC({
p: [{ height: 100 },{ width: 500 },{ color: 0xff00 }]
});
console.log(CSSC("p").first().parse());
/*
p {
height: 100px;
}
*/
```
---
### .last()
**last** is a method to get a Handler object with last style element. This method is equivalent to `.pos(-1)`
```
.last()
```
**`Return value`** - Handler object with last style element
#### Example
```javascript
CSSC({
p: [{ height: 100 },{ width: 500 },{ color: 0xff00 }]
});
console.log(CSSC("p").last().parse());
/*
p {
color: rgb(0, 255, 0);
}
*/
```
---
## Properties
---
### .e
**e** is an Array with CSS Objects
```javascript
CSSC({
".className": {
border: "1px solid #000"
},
".className1": {
border: "1px dotted 0x0"
},
".className2": {
border: "none"
}
});
CSSC(".className").e
```
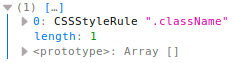
```javascript
CSSC(/\.className/).e
```
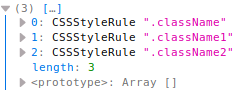
---
### .selector
**selector** is a String / RegEx / Array / ...
If the handler has 1 CSS object, the selector is generally a String.
```javascript
console.log(CSSC("#mySelector").selector);
/*
#mySelector
*/
```